Algorithms
Nth Digit
思路: 没看明白题意,就去抄作业了😰,题意是说当序列中的数字是两位数、三位数等后,第n个就不再是序列中的第n个数,比如10中的1是第10个数字,0是第11个数字。那么需要判断数在序列中的数字是多少,判断是几位数。
1 | 1 1-9 |
参考:
https://www.cnblogs.com/grandyang/p/5891871.html
https://blog.csdn.net/Cloudox_/article/details/53420984
1 | class Solution { |
1 | class Solution { |
Review
本周阅读了关于OSINT的英文文章:
1、Open-Source Intelligence (OSINT) Reconnaissance
3、A Guide to Open Source Intelligence Gathering (OSINT)
一个每周分享OSINT相关资源的博主: https://medium.com/@sector035
Technique
《计算机网络》内容分发网络
内容分发网络(CDN,Content Delivery Network): 在一组分布在不同地点的节点中哪个节点拥有所请求页面的副本,并且指示客户端使用附近的节点作为服务器。
如图,CDN源服务器将一份内容副本分发到CDN中的其他节点。
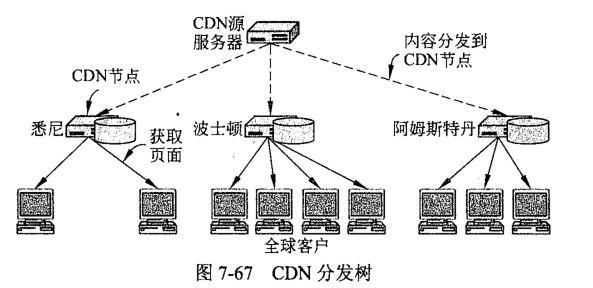
使用树结构有3个好处:
- 内容的分发具备可扩展性。随着越来越多的客户需要,可以使用更多的CDN节点,当CDN节点之间分发变成瓶颈时,可以采用更多的树层次。
- 每个客户都享有不错的性能,因为是从附近的服务器获取页面,建立连接所需要的往返时间越短,TCP慢启动得更快,而且较短的网络路径经过Internet上拥塞区域的可能性较小。
- 放置在网络上的总负荷也保持在最低水平。
支持一颗分发树的另一种简单方法是使用镜像,源服务器将内容复制给CDN节点,不同网络区域的CDN节点称为镜像点。
第三种方法是DNS重定向(DNS redirection)。如图所示:
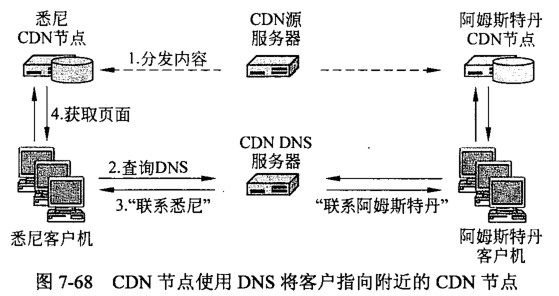
该种方法将客户端映射到某个CDN节点时,至少需要考虑两个因素:
- 网络的距离。客户端应该有一条距离短、容量高的网络路径到达CDN节点。
- CDN节点的当前负载。均衡整个CDN节点的负载非常有必要,应该把可客户端映射到稍微远一点但负载轻一点的节点。
Share
在做项目时,平台中提供了REST Client,第一次玩这个,查了一些相关的资料:
怎样用通俗的语言解释REST,以及RESTful? - 覃超的回答 - 知乎
https://en.wikipedia.org/wiki/Representational_state_transfer