Algorithms
Guess Number Higher or Lower
思路: 二分。
1 | // Forward declaration of guess API. |
Ransom Note
思路: HashMap。
1 | class Solution { |
Review
本周阅读英文文章:
1、Microsoft security integration: Graph Security API to integrate a variety of security solutions
2、Microsoft Defender ATP — MineMeld. Bring Your Own Threat Intelligence feeds
3、Azure Sentinel — MineMeld. Bring Your Own Threat Intelligence feeds
Technique
Tanenbaum《计算机网络》传输层-多路复用
多路复用(或多个会话共享链接,虚电路和物理链路)在网络体系结构的不同层次发挥着作用。在传输层,有几种方式需要多路复用。例如,如果主机只有一个网络地址可用,则该主机上的所有传输连接都必须使用这个地址。当到达了一个段(表示传输实体之间发送的消息,一些老协议使用传输协议数据单元(TPDU, Transport Protocol Data Unit)),必须有某种方式告知它交给哪个进程处理。这种情况,称为多路复用(multiplexing),如图a。
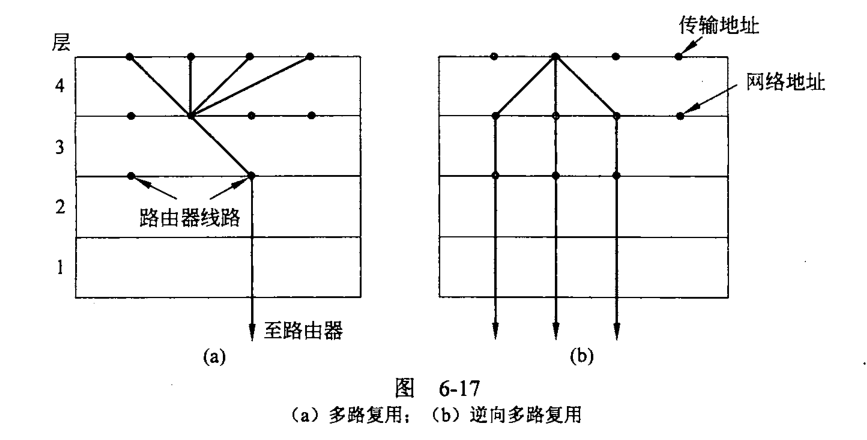
多路复用在传输层非常有用还存在着另一个原因。例如,假设一台主机有多条网络路径可用。如果用户需要的带宽和可靠性比其中任何一条路径所能提供的还要多,那么一种解决办法是以轮询的方式把一个连接上的流量分摊到多条网络路径,这种手法称为逆向多路复用(inverse multiplexing),如图b。若打开了K条网络连接,则有效带宽可能会增长K个因子。逆向多路复用的一个例子就是流控制传输协议(SCTP, Stream Control Transmission Protocol),它可以把一条连接运行在多个网络接口上。相反TCP使用了单个网络端点。逆向多路复用也可以应用在链路层,把几条并发运行的低速率链路当作一条高速率链路使用。
Share
使用smartmontools软件包检查磁盘健康情况
1 | sudo apt install smartmontools |
smartmontools的用户接口是smartctl应用程序。该应用会检测磁盘并报告设备状况,因为smartctl需要访问原始磁盘设备,所以必须以root身份执行。命令输出中有基本信息的标题、原始数据值以及检测结果。标题涵盖了被检测设备的各种细节信息以及该报告的时间戳,原始数据值包括错误计数、准备时间(spin-up time)、加电时间等。
选项-a会报告设备的全部状态信息:
1 | smartctl -a /dev/sda1 |
十分尴尬,我的虚拟机不支持,网上搜索了相关信息,如果是VMware ESXi,那么可以看一下这个文章: https://kb.vmware.com/s/article/2040405